AWS Lambda
Learn how to easily manage environment variables for your AWS Lambda functions.
This guide will show how to sync secrets from Doppler to AWS Lambda functions.
You can also use our AWS Secrets Manager or AWS Parameter Store integrations to sync secrets from Doppler to AWS.
Prerequisites
- Experience deploying Lambda functions using environment variables for configuration
- AWS CLI installed and authenticated
- IAM user with the
lambda:UpdateFunctionConfiguration
andGetFunctionConfiguration
permissions enabled - The jq CLI installed
AWS Permissions
In order to get and set Lambda environment variables, your IAM user will need a policy attached with the following permissions.
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "LambdaConfig",
"Effect": "Allow",
"Action": [
"lambda:UpdateFunctionConfiguration",
"lambda:GetFunctionConfiguration"
],
"Resource": "*"
}
]
}
Import Variables
You will need to import your Lambda environment variables to Doppler before continuing, as the AWS CLI only allows you to replace environment variables. You can do this manually using the Doppler dashboard, or the Doppler CLI locally.
Here is a CLI example using the doppler secrets upload
command, specifying the Doppler project and config, plus the Lambda function name in the --function-name
flag.
# Change the --project, --config and --function-name values for your code
doppler secrets upload --project lambda --config prd \
<(aws lambda get-function-configuration --function-name doppler-test | jq .Environment.Variables)
Service Tokens
To sync your secrets to Lambda as part of a CI/CD job e.g. GitHub Actions, the Doppler CLI requires a Service Token to provide read-only access to a specific config and is exposed to the CLI via the DOPPLER_TOKEN
environment variable. This would normally be provided by the environment, e.g GitHub Secret, but for this guide, we'll provide it manually.
export DOPPLER_TOKEN=dp.st.prd.xxxx
Example Lambda
If you're modifying an existing Lamba, you can skip to the Lambda Secrets Sync section.
You can test Doppler secrets sync with a new Lambda by creating a Lambda function named doppler-test using Node 12.x.
Now change the code to a simple example that returns a config object with two environment variables synced from Doppler.
exports.handler = async function(event, context) {
const config = {
'API_KEY': process.env.API_KEY,
'DB_URL': process.env.DB_URL
}
return config;
}
Then click Deploy.
Now click Test which will ask you to define a test event. We're not using data from the test event so the default *hello-world** template is fine.
Now that our Lambda is set up, create a new project in Doppler named lambda, populating the prd environment with the two secrets required by our code (it doesn't matter what the values are).
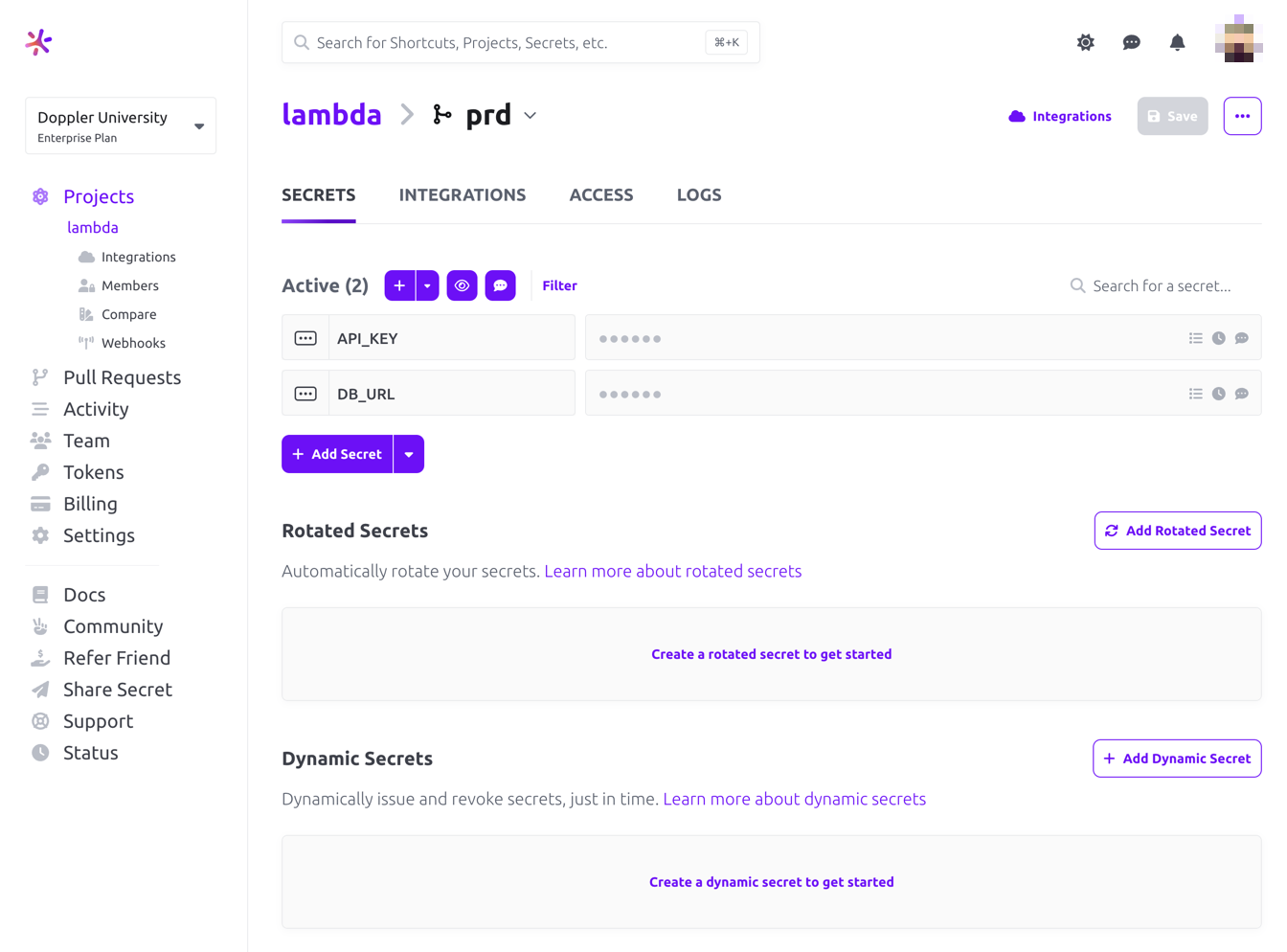
Then click on the Access tab, create a Service Token named Lambda Production and copy the value.
Then manually make the Service Token available to the Doppler CLI.
# This should normally be provided by your CI/CD system
export DOPPLER_TOKEN=dp.st.prd.xxxx
Now you're ready to sync secrets to Lambda!
Secrets Sync
To sync all secrets in a single command, the secrets are downloaded in JSON format and passed to the AWS CLI:
aws lambda update-function-configuration --function-name $FUNCTION_NAME \
--environment "$(doppler secrets download --no-file | jq '{Variables: .}')"
If the command ran successfully, you'll see your secrets and three Doppler config variables in the Environment
object output to the terminal by the AWS CLI.
"Environment": {
"Variables": {
"API_KEY": "6387dc57-25c9-48d4-8969-bc5ba7dc72a2",
"DOPPLER_CONFIG": "prd",
"DB_URL": "postgres.123456789012.us-west-2.rds.amazonaws.com",
"DOPPLER_ENVIRONMENT": "prd",
"DOPPLER_PROJECT": "lambda"
}
}
You can also verify the existence of the environment variables by viewing them in the Lambda console.
If following along with the example Lambda, head go to the Lambda console, click Test and now, you should now see your populated config values in the Execution result section.
Awesome Work!
Now you know to use Doppler to easily sync secrets to AWS Lambda functions in CI/CD environments.
Updated about 2 months ago