Secret Generation
Learn about how to generate secrets from the Doppler dashboard!
For situations where you need a secret value that's not being provided by a third party, you can generate those from right inside your Doppler dashboard using generated secrets!

You can create a Random Value, Symmetric Key, or a Key Pair to use as the value of a secret.
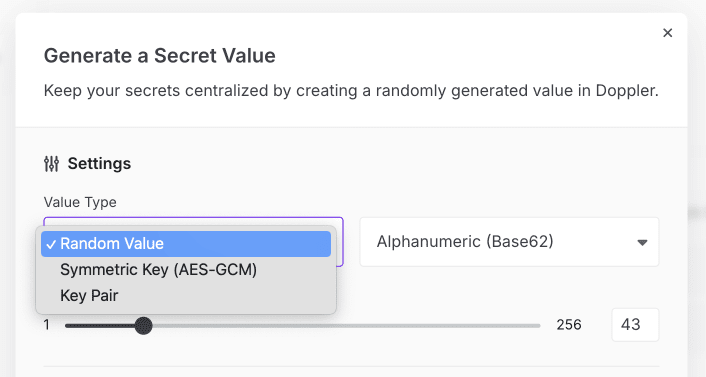
Overview
Value Type | Generated Value |
---|---|
Random Value | Value containing Alphanumeric (Base62), Hex, Base64, or Base64 URL characters |
Symmetric Key | Symmetric AES-GCM key |
Key Pair | ECDSA or ECDH public and private pkcs8 key pair that's base64 encoded |
All secrets are generated using the SubtleCrypto interface of the Web Crypto API.
Random Value
The Random Value option will generate a value for your secret using the selected format.
Formats
The available formats are as follows:
- Alphanumeric (Base62) -
0-9
,a-z
, andA-Z
- Hex (Base16) -
0-9
anda-f
- Base64 -
0-9
,a-z
,A-Z
,+
,/
, and=
- Base64 URL -
0-9
,a-z
,A-Z
,-
,_
, and=
Note that Base64 and Base64 URL formats generate a random secret using those character sets and are not encoding any meaningful data (and thus not meant to be decoded).
Bytes of entropy
You can choose how much entropy is used for your secret. More entropy results in a longer secret. The default is 32 bytes of entropy, which should be enough for most uses, but can be adjusted to as high as 190 bytes if required.

Symmetric Key
The Symmetric Key option will generate an AES-GCM symmetric key (either 256-bit or 128-bit based on what you select).
Key Pair
The Key Pair option creates an ECDSA or ECDH public and private key pair depending on what you select. The key pairs will be in pkcs8 format and will be base64-encoded. These will be stored in two separate secrets, which you're able to name individually.
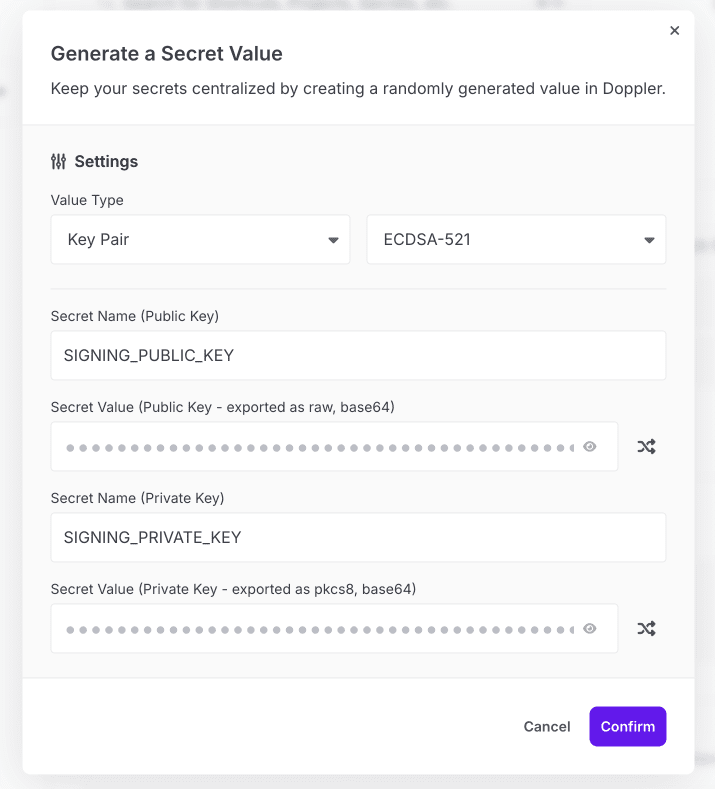
Regenerating Values
Currently, to regenerate these values, you must regenerate from the Private Key secret entry.
Compatibility
These key pairs are generated in-browser using SubtleCrypto. It's not currently possible to generate OpenSSH key pairs in-browser, so the key pair that's generated will not work with openssl
or OpenSSH tools.
Usage
function str2ab(str) {
const buf = new ArrayBuffer(str.length);
const bufView = new Uint8Array(buf);
for (let i = 0, strLen = str.length; i < strLen; i++) {
bufView[i] = str.charCodeAt(i);
}
return buf;
}
const privateKeyBase64 = "MIHuAgEAMBAGByqGSM49AgEGBSuBBAAjBIHWMIHTAgEBBEIAuOQ7wchpXzehkzl6ywqmayBl+Am+TtQ5AkMZDOVPq6AkRN4w1YhLfyCCRWmFgPzfvuQqImN7Ry5bfeqMEN2HVguhgYkDgYYABAGINhz1Z/yOtijOrsw/DDJo+hV2PnzgxzJUsDOUOxAUs+azx6T1TnMzPMSI0mzdZzqdeYMIJooM2euM+ZbrJgmgGgC+r2/kSRIzr4sq4X9X4hGWhRITFX+WiVS+OE0VH549rbzD+prixzON/Ta6scJgc5JjRKO09EwO6Zp/8W/dl7GHJQ==";
const importedPrivate = await window.crypto.subtle.importKey(
"pkcs8",
str2ab(atob(privateKeyBase64)),
{ name: "ECDSA", namedCurve: "P-521" },
true,
['sign']
);
const { subtle } = require('crypto').webcrypto;
(async function() {
const privateKeyBase64 = "MIHuAgEAMBAGByqGSM49AgEGBSuBBAAjBIHWMIHTAgEBBEIAuOQ7wchpXzehkzl6ywqmayBl+Am+TtQ5AkMZDOVPq6AkRN4w1YhLfyCCRWmFgPzfvuQqImN7Ry5bfeqMEN2HVguhgYkDgYYABAGINhz1Z/yOtijOrsw/DDJo+hV2PnzgxzJUsDOUOxAUs+azx6T1TnMzPMSI0mzdZzqdeYMIJooM2euM+ZbrJgmgGgC+r2/kSRIzr4sq4X9X4hGWhRITFX+WiVS+OE0VH549rbzD+prixzON/Ta6scJgc5JjRKO09EwO6Zp/8W/dl7GHJQ==";
const importedPrivate = await subtle.importKey(
"pkcs8",
Buffer.from(privateKeyBase64, "base64"),
{ name: "ECDSA", namedCurve: "P-521" },
true,
['sign']
);
})()
Updated about 2 months ago