Cloudflare Pages
Learn how to sync your Doppler secrets with Cloudflare Pages
This guide is designed to get you set up with syncing your secrets to Cloudflare Pages.
Prerequisites
- You have an account setup at Cloudflare.
- You have a Cloudflare Page created.
Cloudflare API Token
Go to the token creation page in your dashboard, then click on the Create Token button:
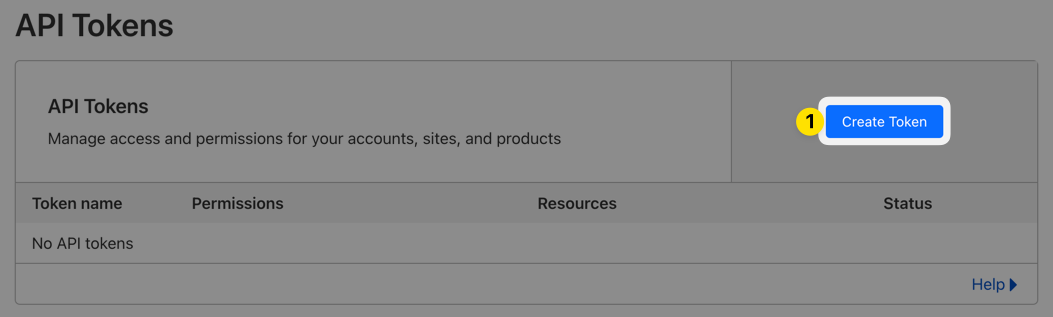
Next, click the Get Started button in the Create Custom Token section:
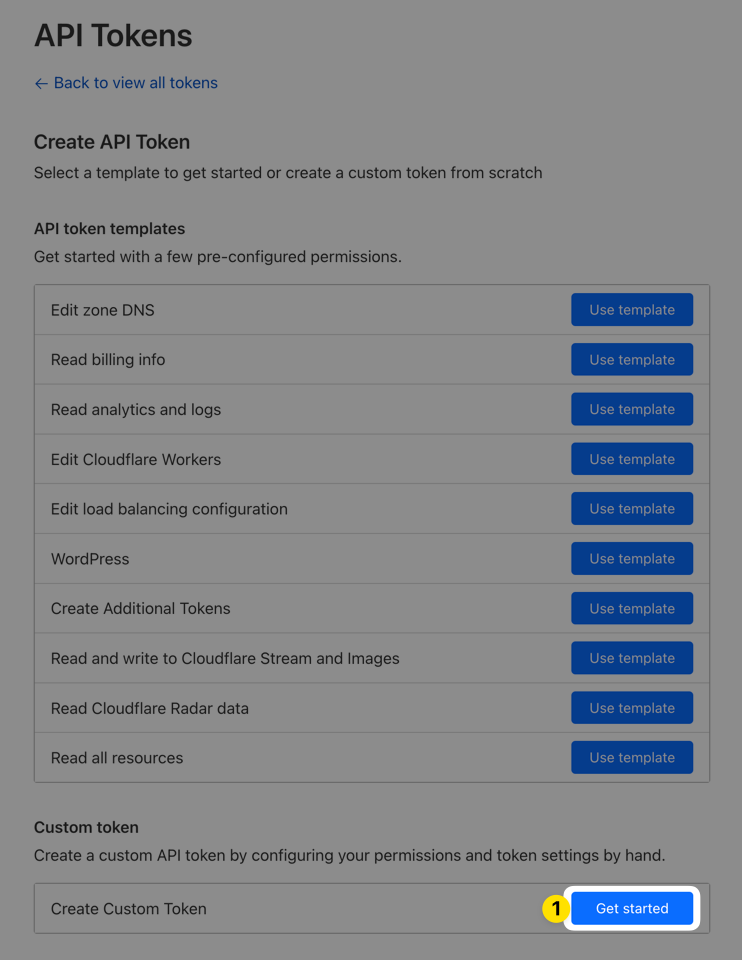
On the resulting form, name your token how you'd like (we use "Doppler" here), add the Account:Cloudflare Pages:Edit and Account:Account Settings:Read permissions, and include any accounts you want to be available as options when setting up syncs, click the Continue to summary button, and finally click the Create Token button:
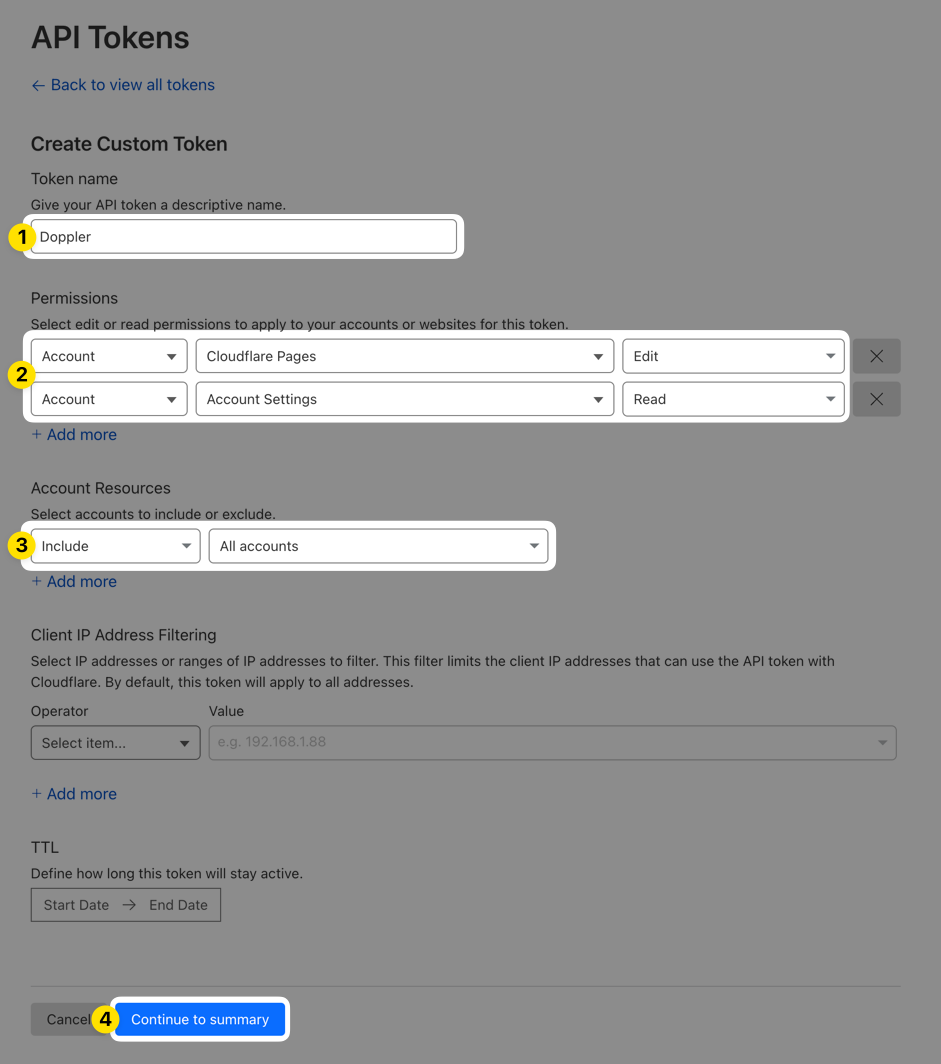
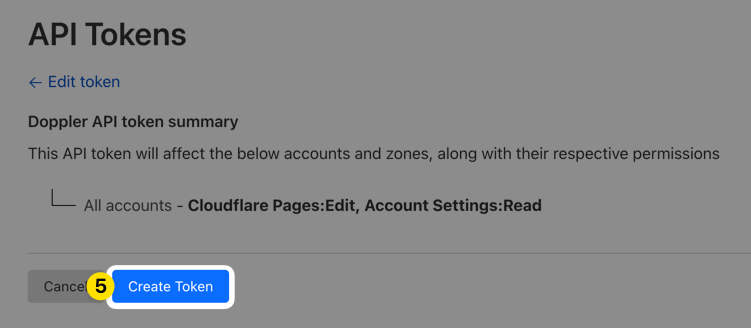
Save the token revealed on the next page to use in the next part of the setup process.
Authorization
In your Doppler project, navigate to Integrations and select Cloudflare Pages:
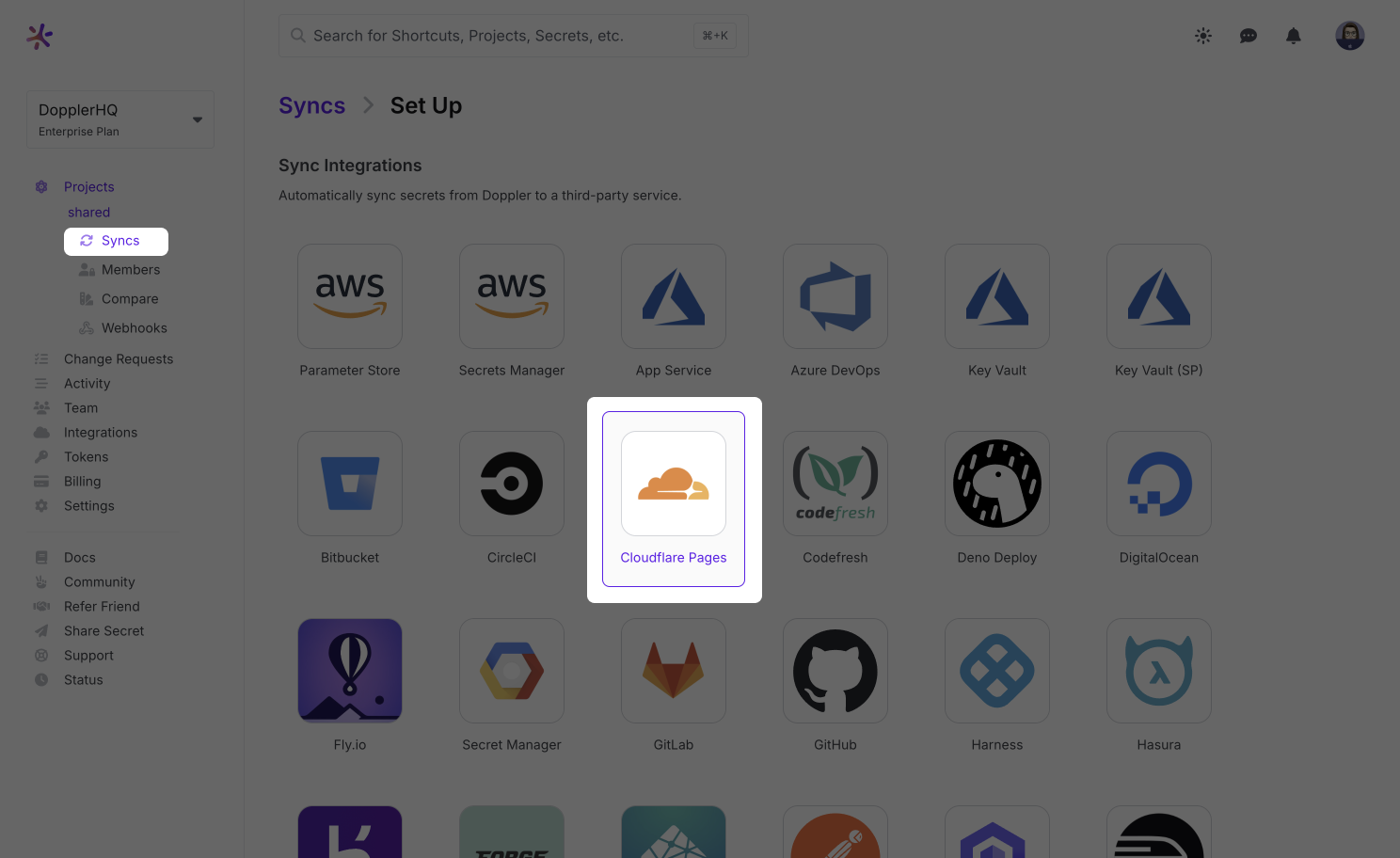
Set the Name to anything you like (this will be used to help you identify this sync when viewing the integrations for this config) and paste the API token you created in the previous step into the API Token field. Then click Connect.
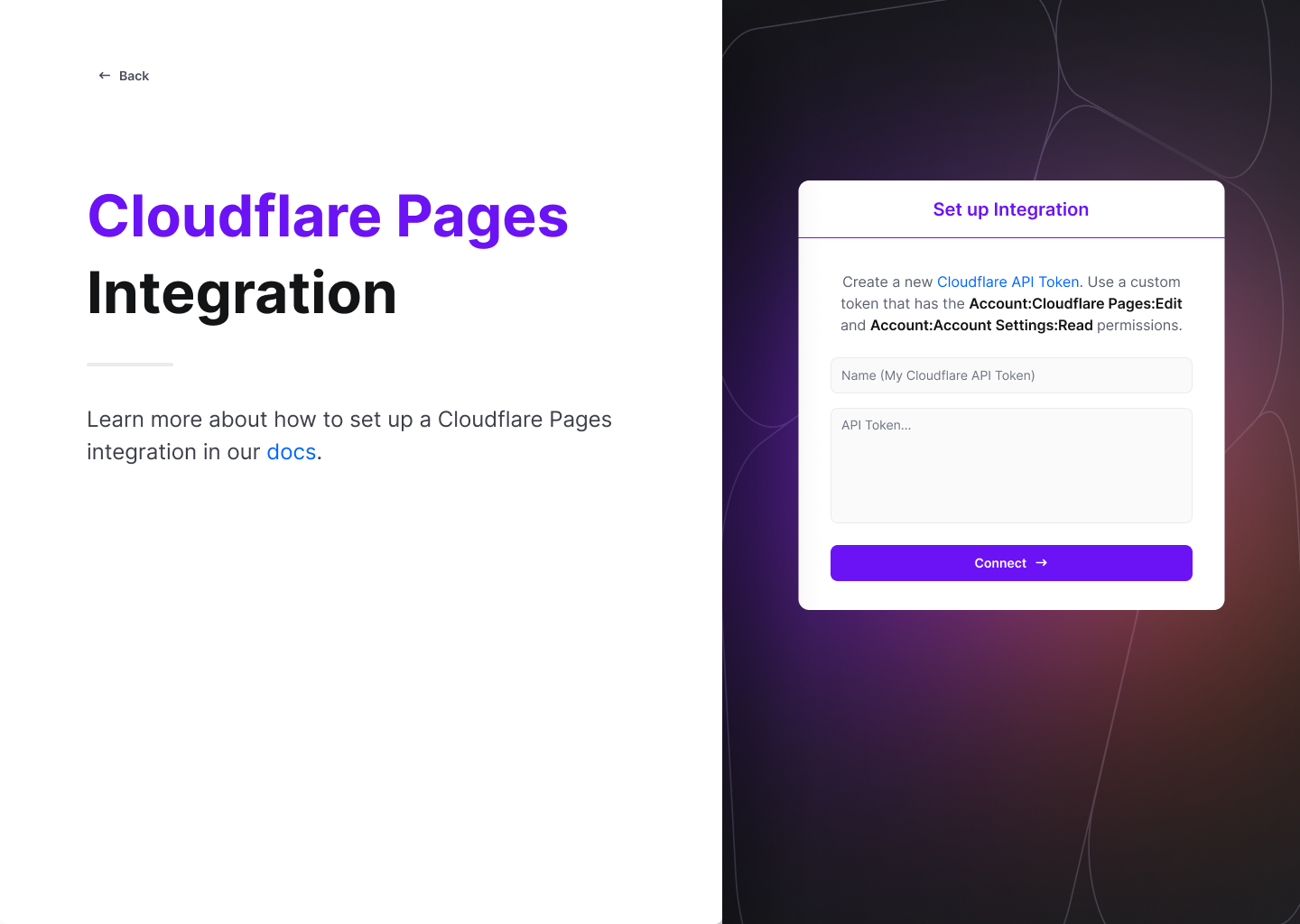
You have now successfully connected your Cloudflare account with Doppler!
Configuration
Next, you can select which Cloudflare account, project, and environment you would like to sync to along with which Doppler config you want to sync over. You can also choose the import behavior for any variables already existing in your Cloudflare project when the first sync is performed.
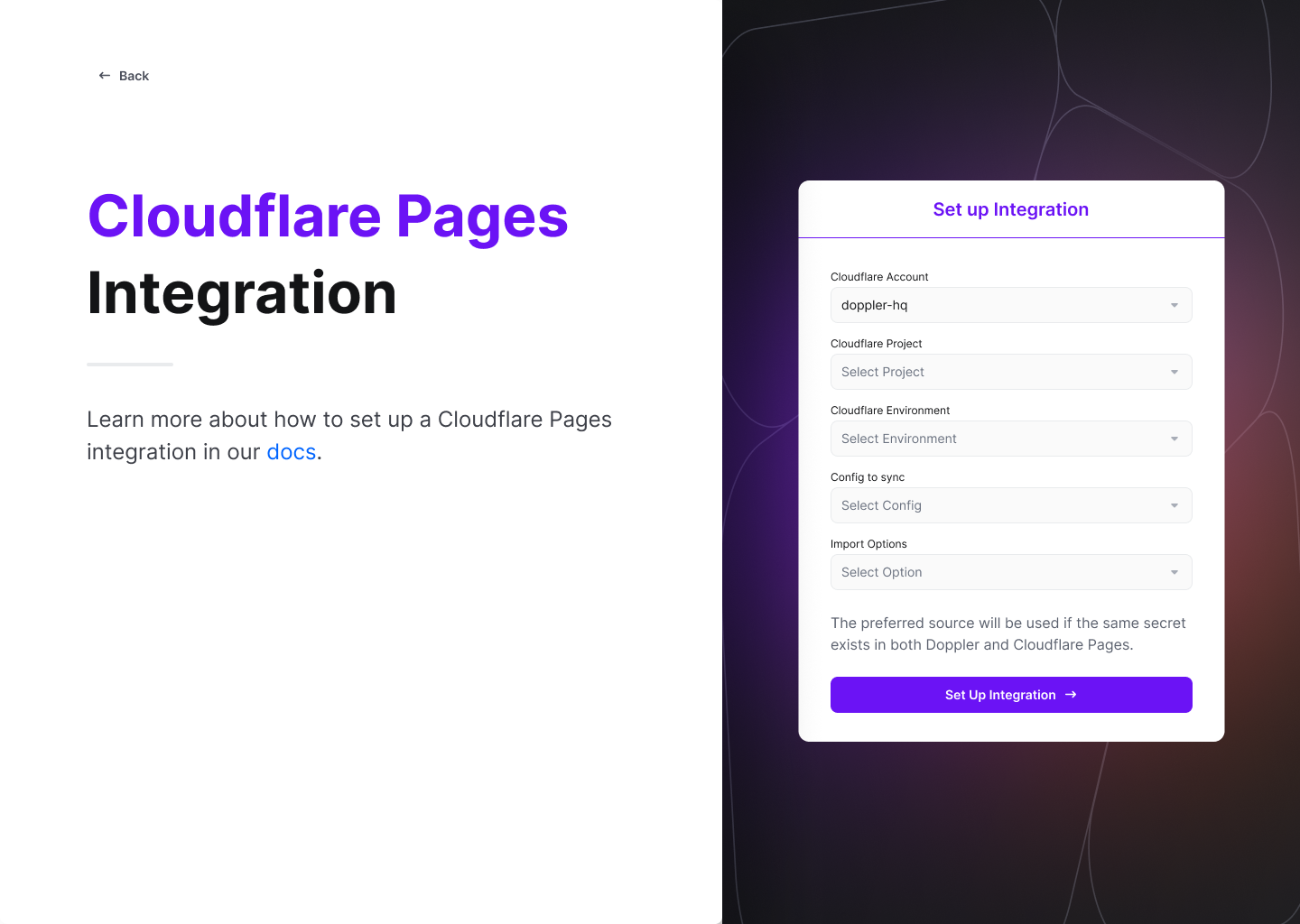
Click Set Up Integration and you're all set!
The secrets from your selected config will be immediately and continuously synced with Cloudflare.
Amazing Work!
Now you are all set up on Cloudflare. The next time you deploy your secrets will be fetched from Doppler.
Updated 3 days ago